(4) Control - An example interface using the Megabot Robot
As we described in our previous tutorial on building and loading kernel modules on the Qualcomm Robotics RB5, the Ubuntu 18.04 installation that is part of the Qualcomm Robotics RB5 LU build includes minimal package support to reduce OS complexity. With this in mind, if we wish to install custom drivers, we need to perform the process described. Thankfully, if you have build and loaded the kernel modules described in our tutorial, you will be able to interface with a standard joystick controller over USB and serial over USB.
With the preliminaries completed, we will use the Megabot Robot as an example to interface with an Qualcomm Robotics RB5. In this case, we will use the megapi
Python module designed for the Megabot to communite with the robot. This can be readily installed using pip install megapi
.
Here we define a set of primitive actions that can control this four-mechanum-wheel robot. The set of primitive control actions include move left
,right
,forward
, in reverse
, rotate clockwise
, counter-clockwise
, and stop
. Yes, it come as a surprise to many but the wheels on the robot contain a number of different rollers that ultimately influence the kinematics of the robot and jointly provide very interesting properties such as rotating in place and moving sideways!
The inverse kinematics of the robot can be described below.
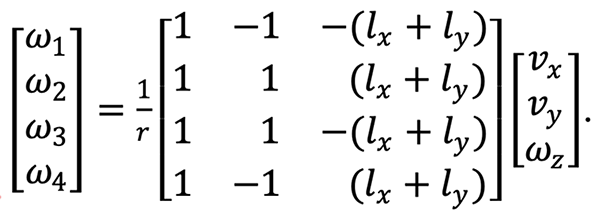
The following code snippet implements the control conditions for each of the actions defined.
1 | def move(self, direction, speed): |
This script has been implemented using ROS1 and ROS2. While the robot can be controlled using the standard joy
node using an USB jostick controller, it can additionally be controlled a standard ROS message for higher level planning strategies. The process of building and running the ROS nodes is outlined below.
ROS1
Create a workspace, clone the ROS1 implementation, and build the package. Make sure ROS is in your path, i.e. source /opt/ros/melodic/setup.bash
.
1 | mkdir -p rb5_ws/src && cd rb5_ws/src |
Start the control node
1 | ros run rb5_control rb5_mpi_control.py |
ROS2
Create a workspace, clone the ROS2 implementation, and build the package. Make sure ROS is in your path, i.e. source /opt/ros/dashing/setup.bash
.
1 | mkdir -p rb5_ws/src && cd rb5_ws/src |
Start the control node
1 | ros2 run rb5_ros2_control rb5_mpi_control.py |